This topic is all about how you can put your PHP code into separate source files and use those functions in your event scripts in PHPRunner applications. When your applications start to get more complicated, a few things happen.
a) you start to get longer and more complicated event scripts, and in my personal opinion, once you are over a few dozen lines of PHP, you will probably do better structuring your scripts into functions.
b) you start to get script features you want to include in more than page or button, or even in more than one PHPRunner application – having your code in a separate PHP file makes this trivial and means you aren’t duplicating code.
So how can an external PHP file get access to PHPRunner and application session variables, the PHPRunner data access layer (DAL), and the connection object? You certainly want to be able to do all the things in your external file that you could in the event editor itself.
If you haven’t tried this yet, It’s really quite easy to do, and for the right applications, it’s very important to do.
Let’s say I want to add a button on a parameter screen (such as an Add screen – I’ll cover how to do user parameter input screens in another blog sometime soon!) and the user puts in a start-date / end-date for report/process parameters, clicks the button, and now we need to actually do the report.
Here’s how we architect that.
Calling the PHP external functions in an event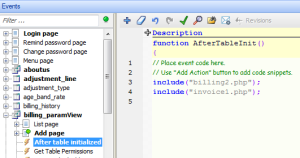
In my example, I am using the Custom Add event to intercept the regular “Add” processing… and that way I can use the add page fields as a parameter input screen, and discard the add. During the CustomAdd event, I’m going to put some code in to call the functions in the external PHP file and pass the PHPRunner connection and any other variables needed to get the job done.
You can see that in my example, I put my external file “include” statements in the After Table Initialized event. This means the functions in the two external files will be available to all of the events in the Add Page.
Where to put the External PHP files?
You put the actual files in the PHPRunner project sub-folder called “source”. typically that folder is empty. But if you put your source file in the “PHPRunnerProjects/myappname/source” folder, then PHPRunner will include it in the build and output folder deployment automatically.
You can of course locate your source files anywhere, but I find the above to be easy.
Calling the External functions
Most importantly, getting variables and environment to those PHP functions so that they work as part of the PHPRunner model.
For the above example, I put the following into my CustomAdd event.
// Place event code here. // I want to pass the globals/session parms and connection object to the exernal files so…. global $conn; //first generate the invoice lines... // main function in billing2.php f_run_billing($conn, $values['billing_date'], $values['billing_period'], $values["employer_id"]); // main function in invoice1.php: wrap them in a pdf... f_print_billing($conn, $values['billing_date'], $values['billing_period'], $values["employer_id"]); $var = "Billing Processing is Complete!"; echo "<script>alert('$var')</script>";
In the External PHP File
the only thing to be aware of is that it has to be a proper PHP file, with opening <?PHP and closing ?> PHP script tags. everything else is standard PHP. You can use the sessions variables, that PHPRunner uses, and pretty much anything else. If you wanted to use the Data Access Layer (DAL) for example, you would declare it as a global in your event like
global $dal;
and pass that as a parameter object to the external PHP file and you’d be able to code just like you were in the event script itself.
<?PHP function f_run_billing($connection, $param_rundate, $param_billing_period, $arg_employer_id){ … … your code… } ?>
Hope that all makes sense! Happy code organizing!
paul
dear paul
want to know sending sms from selected records in phprunner 6.2 using ozeki sms server gateway same concept to send selected user email button on list page
did u knw how to do that
Hi Ahsun, which bit are you having issues with? the PHPRunner loop control for processing selected records should be pretty much identical to the email sending examples that xlinesoft publish. Put a button in the button area above your list grid (where you see delete selected etc). In the button event, PHPserver side put:
global $dal;
while ( $data = $button->getNextSelectedRecord() ) {
// do something with each record found…
$ct = $ct + 1;
$sql = “Update some_table set some_field = 1 where id = “.$data[“id”];
CustomQuery($sql);
}
$result[“txt”] = $ct . ” records were updated.”;
In the javascript after_client event you can put some sort of “done” message
var message = result[“txt”];
window.parent.location=”adjustment_line_list.php?a=return”;
ctrl.setMessage(message);
You would clearly want to do some SMS message construction and then call the ozeki gateway to send your SMS. I have never used Ozeki server. For sending SMS messages there is a free and easy way, and all you do is send an email to the phone number at the carrier’s domain. e.g., if you want to send an SMS to 555-123-1234 and the cell subscriber is on Verizon, you would just send an email to 5551231234@vtext.com.
All the carriers have a similar capability. This blogger http://20somethingfinance.com/how-to-send-text-messages-sms-via-email-for-free/ put a nice list of the carrier addresses together.
Xlinesoft also put together a good tutorial on interfacing to Twilio to do the same job. you can find it at http://www.asprunner.com/forums/topic/22786-sending-record-data-via-sms/
hope that helped you a little. Have fun!
paul